In this tutorial we will see how to configure an ASP.NET Core 3.1 web application with React as front end and Secure User membership implementation using Identity Server 4 using MySQL database.
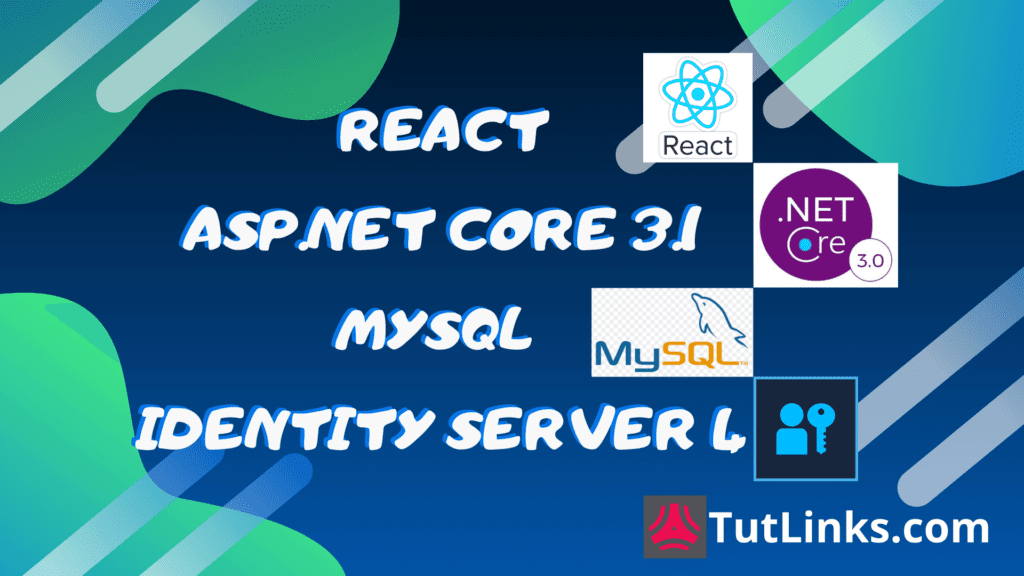
Table of Contents
- PreRequisites
- Steps to Configure Identity Server 4 using MySQL in ASP.NET Core 3.1 React Web application
- Delete Data Directory
- Install Pomelo.EntityFrameworkCore.MySql
- Create MySQL database
- Update appsettings.json to point to MySQL Connection String
- Update Startup.cs to use MySQL
- Scaffold New Migration and Update MySQL Database
- Build and Run the ASP.NET Core 3.1 React Web with Identity Server 4 using MySQL
- Check the MySQL database for the registered user
- Video Tutorial
PreRequisites
- Microsoft Visual Studio 2019 Community Edition
- .net core 3.1 https://dotnet.microsoft.com/download/dotnet-core
- MySQL Tools for Visutal Studio
- mysql-for-visualstudio-1.2.9.msi, download from here and install
- Windows 10 PC
- A browser preferably Chrome, Firefox or IE 11 or higher
Steps to Configure Identity Server 4 using MySQL in ASP.NET Core 3.1 React Web application
Delete Data Directory
Locate and delete the Migrations directory which is found under the Data directory of the solution
Install Pomelo.EntityFrameworkCore.MySql
Right click on the project and select the menu option Manage NuGet Packages. You can find three menu tabs Browse, Installed,Updates. Under the Installed tab, locate and uninstall the package Microsoft.EntityFrameworkCore.SQLServer.
Now navigate to the browse tab and search for and install the package Pomelo.EntityFrameworkCore.MySql
Create MySQL database
Access the MySQL database via MySQL workbench or within Visual Studio by navigating to Server Explorer and connecting to MySQL database server. Now create a database in MySql by running the following MySQL statement.
CREATE SCHEMA reactmembership;
Update appsettings.json to point to MySQL Connection String
Update value of DefaultConnection in appsettings.json to
server=localhost;port=3306;database=reactmembership;user=root;password=p@$$w0Rd;CharSet=utf8
The appsettings.json file will look like this once we have MySQL connection string updated for DefaultConnection
{ "ConnectionStrings": { "DefaultConnection": "server=localhost;port=3306;database=reactmembership;user=root;password=p@$$w0Rd;CharSet=utf8" }, "Logging": { "LogLevel": { "Default": "Warning" } }, "IdentityServer": { "Clients": { "ReactMembershipDemo": { "Profile": "IdentityServerSPA" } } }, "AllowedHosts": "*" }
Update Startup.cs to use MySQL
In Startup.cs file update ConfigureServices method to replace UseSqlServer with UseMySql
public void ConfigureServices(IServiceCollection services) { services.AddDbContext<ApplicationDbContext>(options => options.UseMySql( Configuration.GetConnectionString("DefaultConnection"))); services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); services.AddIdentityServer() .AddApiAuthorization<ApplicationUser, ApplicationDbContext>(); services.AddAuthentication() .AddIdentityServerJwt(); services.AddControllersWithViews(); services.AddRazorPages(); // In production, the React files will be served from this directory services.AddSpaStaticFiles(configuration => { configuration.RootPath = "ClientApp/build"; }); }
Scaffold New Migration and Update MySQL Database
In Visual Studio 2019, Navigate to Tools-> Nuget Package Manager -> Package Manager Console
In Visual Studio, use the Package Manager Console to scaffold a new migration and apply it to the database:
Add-Migration [migration name of your choice] Update-Database
Build and Run the ASP.NET Core 3.1 React Web with Identity Server 4 using MySQL
Build the application using the command Ctrl+Shift+B and hit function key F5 to run the application.
Once the application runs in a browse on localhost, you will see a header menu options to Register. Navigate to Register and provide email and password with confirm password.
Check the MySQL database for the registered user
Verify the user created in MySql database in aspnetusers table by running the following select statement
select * from aspnetusers
You should find the user with email id provided while registering with the web application.
Congratulations 🎉, you have successfully configured the ASP.NET Core 3.1 web app with Secure Membership to use MySQL as the database.
Video Tutorial
You can also go through the detailed video that walks you through configuring ASP.NET Core 3 React SPA web application with Secure Membership that talks to MySQL database.